Switching Gear, move on to a tutorial!
I decided to follow a guide after day01 because I realized that I did not know where to start. Like any sane person, I decided to follow a guide 😃 I will begin using this document: https://gbdev.io/pandocs/The_Cartridge_Header.html.
The first task I chose was to somehow load the cartridge and read data from it. As a result, I wrote a test and worked it out piece by piece.
var assert = require("assert");
var path = require("path");
var Cart = require("../src/core/cartridge.js").default;
describe("Cartridge Loading", function() {
it("Cart should load tetris rom", async function() {
var cart = new Cart();
await cart.load(path.resolve("roms/tetris.gb"));
assert.equal(cart.title, 'TETRIS000000000', "Title is not TETRIS000000000");
assert.equal(cart.manufacturerCode, '\x00\x00\x00\x00', "Manufacturer code is not 0x00000000");
assert.equal(cart.romSize, 0x0, "ROM size is not 32kb: 0x0 option");
});
});
With the test above, I was able to implement the cartridge quite easily Check out the implementation here. Everything was straightforward: Load the rom file into an 8-bit array Read the rom file and output title, licensee code, etc What to do next?
I will write the main GameBoy file- just a GameBoy class with the function run() built-in which will serve as a starting point to run the program. It's time to write another test.
const GameBoy = require('../src/gameboy.js').default;
const path = require('path');
describe('GameBoy', () => {
it('should run the game', () => {
const gameBoy = new GameBoy();
gameBoy.run(path.resolve("roms/tetris.gb"));
});
});
And of course, I like fancy stuff, so I added a logger library.
const pino = require('pino');
const path = require('path');
const transport = pino.transport({
targets: [
{
target: 'pino/file',
options: {
destination: path.resolve('logs/processor.log'),
mkdir: true
}
},
{
target: 'pino-pretty',
options: {
colorize: true,
destination: 1
}
}
]
});
const logger = pino(transport);
And now, logging is a thing.
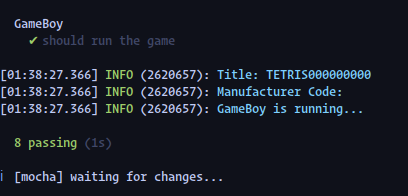
The joy of TDD when it works.
And that marks the end of day02.
Check list
- [x] Prepare cartridge
- [x] Cartridge loaded
- [ ] Checksum
- [x] Stud CPU class
- [ ] Stud PPU class
- [x] Emu class to run the gameboy